Introduction: Writing a Windows Service
Hi, and welcome to this video on writing a Windows service. In the previous video, we explored what Windows services are and how to use related tools. Now, we’ll go through writing a minimal Windows service that can be started and stopped, then gradually add more functionality.
Setting Up the Project
- Create a Console Application:
- Open Visual Studio and start a new project.
- Choose a console application and name it, e.g.,
SimpleSVC
.
- Turning a Console Application into a Service:
- Include the necessary Windows APIs.
- Use
StartServiceControlDispatcher
to register services in the process.
Defining the Service Table
Create an array with entries for the service names and their corresponding main functions:
- Service Name: Define a global variable for reuse.
- Service Entry Function: Define the main function for the service, which will be called by the Service Control Manager (SCM).
Main Service Function and Control Handler
- Service Main Function: This function is invoked by
StartServiceControlDispatcher
. It registers a control handler, which receives commands (e.g., stop, pause). - Control Handler:
- Use
RegisterServiceCtrlHandler
to register a function (e.g.,SimpleHandler
) that handles service commands. - Implement a status reporting system using
SetServiceStatus
to communicate the service’s current state.
- Use
- Service Initialization:
- Set the service status to
SERVICE_START_PENDING
during initialization. - Once initialized, set the status to
SERVICE_RUNNING
.
- Set the service status to
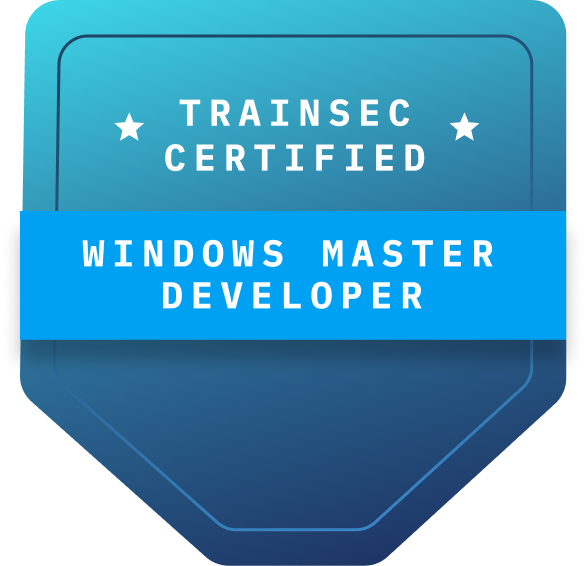
$1,478
$1182 or $120 X 10 payments
Windows Master Developer
Takes you from a “generic” C programmer to a master Windows programmer in user mode and kernel mode.
Testing the Basic Service
- Compile the Code: Ensure the code compiles without errors.
- Register and Start the Service: Use tools like SC or PowerShell to create and start the service.
- Verify Service Functionality: Check if the service starts and stops as expected in the Services applet or Task Manager.
Adding the Logging Functionality
- Create a Mailslot for Logging: Mail slots provide a simple way for client applications to send messages to the service.
- Open a Log File: Use a file to store log messages from the mail slot. Ensure it has exclusive access to avoid conflicts.
- Define the Log Message Structure: Create a structure to hold log levels (e.g., critical, warning, info) and the message text.
Implementing Mail Slot Listening
- Mailslot Initialization: Use
CreateMailslot
to create a mail slot that accepts messages from clients. - Listening for Messages: Use
ReadFile
to retrieve messages from the mail slot and write them to the log file. - Timestamp Logging: Record the date and time of each log entry for easier tracking.
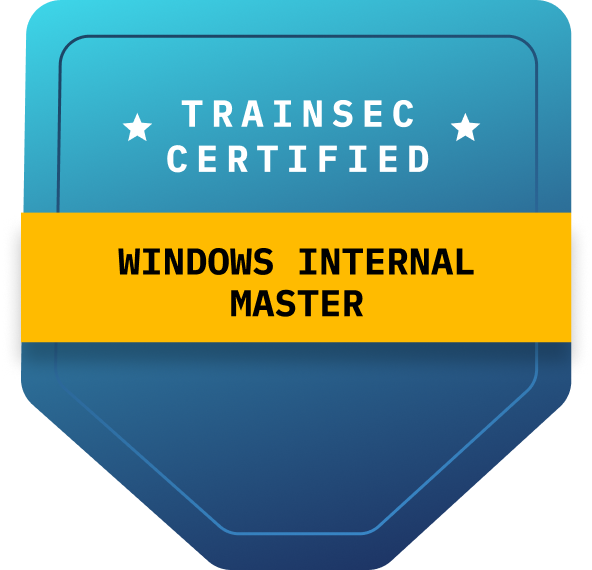
$1300
$1040 or $104 X 10 payments
Windows Internals Master
Broadens and deepens your understanding of the inner workings of Windows.
Setting Up a Client Application
- Create a Client Application: This application will connect to the mail slot and send log messages to the service.
- Format Messages: Structure the messages with process and thread IDs to differentiate log sources.
- Sending Messages: Open the mail slot and use
WriteFile
to send messages to the service.
Addressing Security and Permissions
- Mailslot Security: Configure the security descriptor to allow non-admin users to send messages to the mailslot.
- File Sharing: Adjust file sharing settings to allow reading of the log file even when the service is running.
Troubleshooting and Testing
- Handle Access Errors: Address any “Access Denied” errors by setting appropriate permissions.
- Verify Logging: Check that the log file records messages as expected and allows concurrent access.
- Test Service Restart and Shutdown: Ensure the service stops and restarts cleanly, releasing resources like file handles and the mailslot.
Conclusion
This video demonstrated creating a Windows service, making the service provide logging functionality. This setup provides a foundation for more advanced service features. Play around with the code and experiment with extending functionality, like adding a custom API for logging. You can find the code on GitHub.